C++ Virtual Functions Question:
What is general form of pure virtual function? Explain?
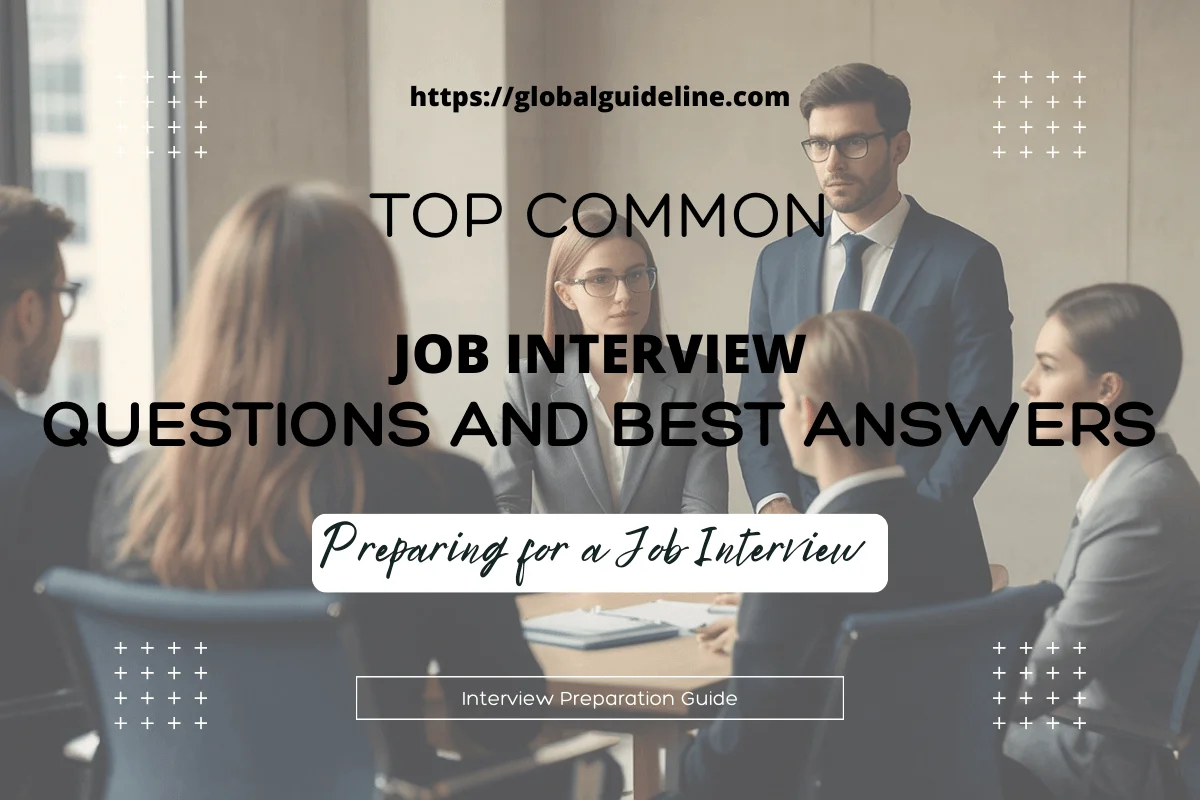
Answer:
A pure virtual function is a function which has no definition in the base class. Its definition lies only in the derived class ie it is compulsory for the derived class to provide definition of a pure virtual function. Since there is no definition in the base class, these functions can be equated to zero.
The general form of pure virtual function is:
virtual type func-name(parameter-list) = 0;
Consider following example of base class Shape and classes derived from it viz Circle, Rectangle, Triangle etc.
class Shape
{
int x, y;
public:
virtual void draw() = 0;
};
class Circle: public Shape
{
public:
draw()
{
//Code for drawing a circle
}
};
class Rectangle: public Shape
{
Public:
void draw()
{
//Code for drawing a rectangle
}
};
class Triangle: public Shape
{
Public:
void draw()
{
//Code for drawing a triangle
}
};
Thus, base class Shape has pure virtual function draw(); which is overridden by all the derived classes.
The general form of pure virtual function is:
virtual type func-name(parameter-list) = 0;
Consider following example of base class Shape and classes derived from it viz Circle, Rectangle, Triangle etc.
class Shape
{
int x, y;
public:
virtual void draw() = 0;
};
class Circle: public Shape
{
public:
draw()
{
//Code for drawing a circle
}
};
class Rectangle: public Shape
{
Public:
void draw()
{
//Code for drawing a rectangle
}
};
class Triangle: public Shape
{
Public:
void draw()
{
//Code for drawing a triangle
}
};
Thus, base class Shape has pure virtual function draw(); which is overridden by all the derived classes.
Previous Question | Next Question |
Do you know what are pure virtual functions? | Can you please explain the difference between static and dynamic binding of functions? |