C++ Friend Question:
What are the C++ friend classes? Explain its uses with an example?
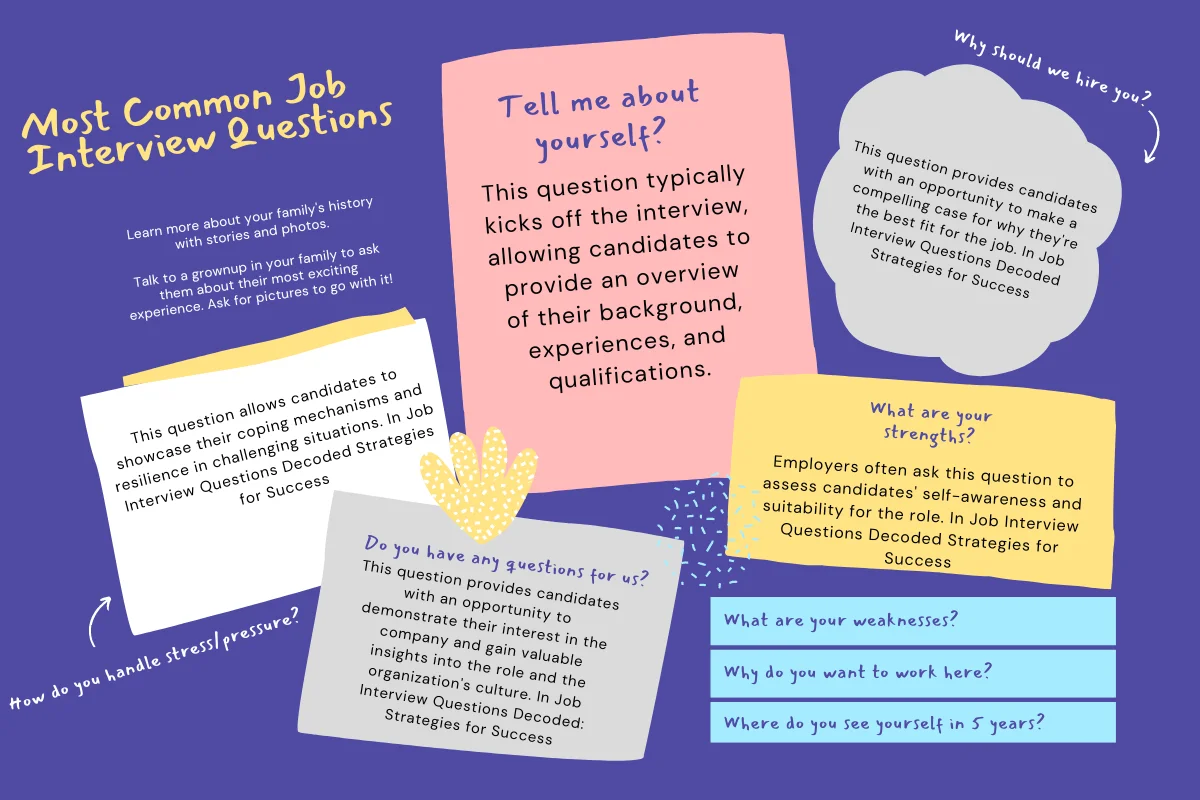
Answer:
A friend class and all its member functions have access to all the private members defined within other class.
#include <iostream>
using namespace std;
class Numbers
{
int a;
int b;
public:
Numbers(int i, int j)
{
a = i;
b = j;
}
friend class Average;
};
class Average
{
public:
int average(Numbers x);
};
int Average:: average(Numbers x)
{
return ((x.a + x.b)/2);
}
int main()
{
Numbers ob(23, 67);
Average avg;
cout<<"The average of the numbers is: "<<avg.average(ob);
return 0;
}
Note the friend class member function average is passed the object of Numbers class as parameter.
#include <iostream>
using namespace std;
class Numbers
{
int a;
int b;
public:
Numbers(int i, int j)
{
a = i;
b = j;
}
friend class Average;
};
class Average
{
public:
int average(Numbers x);
};
int Average:: average(Numbers x)
{
return ((x.a + x.b)/2);
}
int main()
{
Numbers ob(23, 67);
Average avg;
cout<<"The average of the numbers is: "<<avg.average(ob);
return 0;
}
Note the friend class member function average is passed the object of Numbers class as parameter.
Previous Question | Next Question |
List the characteristics of friend functions? | List the advantages of using friend classes? |